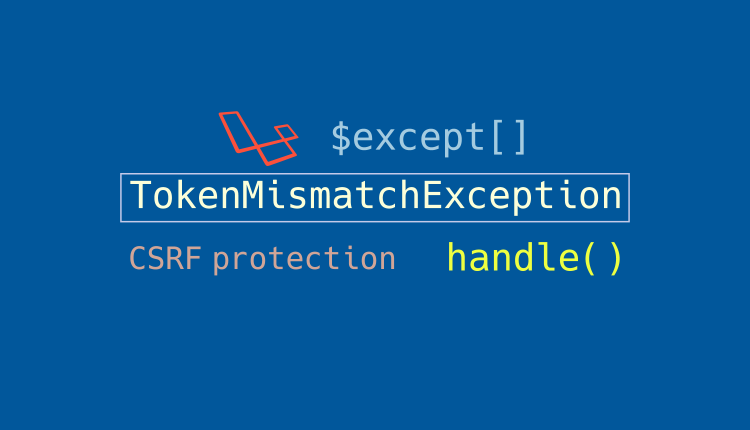
If you stay on one form for too long or you are using some third-party APIs, you get TokenMismatchException. Therefore, you want to get rid of CSRF protection for certain routes.
Table of Contents
Removing middleware
Now, there are a lot of options. First one is to remove VerifyCsrfToken
middleware from web
middlewareGroups
. But this will remove CSRF protection from your entire application. It is not recommended as it makes your application vulnerable to cross-site-request-forgery attack.
Using $except array
Another option that is very popular is to add route path in $except
array in VerifyCsrfToken
class located in app/Http/Middleware/VerifyCsrfToken.php
. Maybe you want to remove CSRF protection for logout page. If you stay on that page for a long time and then click log out, it will return TokenMismatchException. You can simply add /logout
to the $except
array.
protected $except = [ '/logout' ];
It will remove CSRF protection for the /logout
route.
Using a Handle method
Another option that is unknown to many laravel users is to use a handle
method to write some logic to avoid CSRF protection for some routes. Let’s say you want to remove CSRF protection for all routes that starts with api/
. You can do that by visiting VerifyCsrfToken
class located in app/Http/Middleware/VerifyCsrfToken.php
and adding a handle
method. You can simply let the request move forward if it hits routes you want to be spared. Here is how you can do it.
public function handle($request, Closure $next) { if ($request->is('api/*')) { return $next($request); } return parent::handle($request, $next); }
if the request starts with api/
, it is excluded from protection. Otherwise, we simply call the parent::handle($request, $next)
and let the CSRF protection handled as before. You can add as many conditions as you want.
You can learn more about CSRF protection in laravel docs.