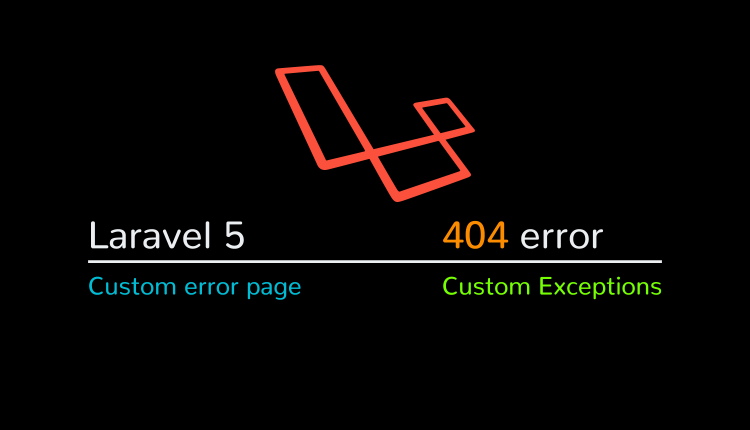
In this tutorial, we will see how to create custom 404 page in Laravel.
PHP Exceptions are similar to the exceptions in other programming languages. Exceptions are thrown when an error or an unknown event occurs. All PHP Exceptions extends the base Exception class.
Laravel provides app/Exceptions/Handler.php class that checks for all exceptions thrown in the application. Basically, every exception thrown in the application can be configured in this file and an appropriate response can be generated.
In this tutorial, we will see how to create a custom response for a 404 page. We are using Laravel 5.6. By default, Laravel returns a Whoops page back. We will see how to return a custom page depending on the exception. This will help you return a custom page for every HTTP Exception you want.
When someone hits a route that is not present, your site shows an error. It affects your SEO as well.
So, let’s see how we can manage 404 page, other HTTP exceptions, and Custom Exceptions in Laravel.
Table of Contents
Setup Laravel Project
Let’s create a new laravel project. Run the following command to create a new project in a folder named errors.
composer create-project --prefer-dist laravel/laravel errors
Handling exceptions
All of the changes related to handling exceptions will be done in app/Exceptions/Handler.php. We will return a view when an error comes.
Custom 404 Page
Let’s create a custom 404 page in Laravel. In the app/Exceptions/Handler.php file, modify the render method.
/** * Render an exception into an HTTP response. * * @param \Illuminate\Http\Request $request * @param \Exception $exception * @return \Illuminate\Http\Response */ public function render($request, Exception $exception) { if ($this->isHttpException($exception)) { if ($exception->getStatusCode() == 404) { return response()->view('errors.' . '404', [], 404); } } return parent::render($request, $exception); }
In the render method, we check if the exception is an HTTP exception. It is important because we are calling getStatusCode() method which is only available on HTTP exceptions. If the status code is 404, we return a view errors.404 with a status code as well. You can change the name of the view if you want.
We have to create a view for the 404 page. So, create a new view file errors/404.blade.php.
<!DOCTYPE html> <html> <head> <title>Page not found - 404</title> </head> <body> The page your looking for is not available </body> </html>
If you want to create a custom page for any other HTTP exception, simply add a new if statement and change 404 with the new status code. Here’s the render method for handling 404 and 500 status code custom pages.
public function render($request, Exception $exception) { if ($this->isHttpException($exception)) { if ($exception->getStatusCode() == 404) { return response()->view('errors.' . '404', [], 404); } if ($exception->getStatusCode() == 500) { return response()->view('errors.' . '500', [], 500); } } return parent::render($request, $exception); }
Here’s the 404 response from our application.
Custom HTTP Exceptions pages
We can also automate this process. It would be great if we only have to create a new view file for the exception. The need to add code to the app/Exceptions/Handler.php file for every HTTP exception will no longer be required. In the app/Exceptions/Handler.php modify the render method.
public function render($request, Exception $exception) { if ($this->isHttpException($exception)) { if (view()->exists('errors.' . $exception->getStatusCode())) { return response()->view('errors.' . $exception->getStatusCode(), [], $exception->getStatusCode()); } } return parent::render($request, $exception); }
Now, you are only required to create a view with the status code of the exception. render method will check if the view exists with the status code of the exception. If the view is present, it will simply return an HTTP response with that view and the status code.
For Example, we can create errors/404.blade.php for 404 error pages, errors/500.blade.php for 500 error pages and so on.
Handling Custom Exceptions
Let’s first create a custom exception. Run the following code to create an exception named TestingHttpException.
php artisan make:exception TestingHttpException
In the app/Exceptions/Handler.php file, modify the render method.
public function render($request, Exception $exception) { if ($exception instanceof TestingHttpException) { return response()->view('errors.testing'); } return parent::render($request, $exception); }
If the exception is an instance of TestingHttpException, it will return errors.testing view.
You can also use the abort method with 404 code and it will serve an HTTP response with the custom 404 page.
You can use these techniques for handling all types of exceptions.
Learn more about Laravel:
Laravel Auth: Login With Email Or Username In One Field
Sending Notifications to Telegram Messenger with Laravel