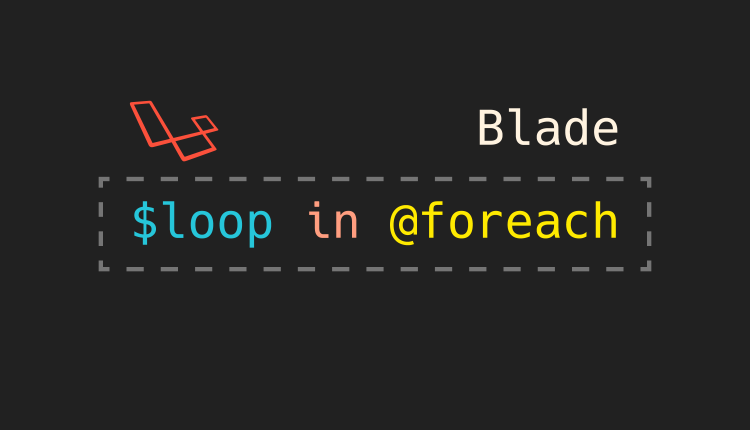
Laravel blade has a foreach directive that we can use the same way as we use the foreach loop in PHP. @foreach
directive is more powerful than a normal foreach loop because of the $loop
variable that is available inside every $foreach
loop.
The $loop
variable is a stdClass
object and it provides access to useful meta information about your current loop. $loop
variable exposes eight useful properties.
$loop->index
Returns a 0-based current loop iteration; 0 would mean the first iteration$loop->iteration
Returns a 1-based current loop iteration; 1 would mean the first iteration$loop->remaining
Number of iterations remaining in the loop; if there are a total of 10 iterations and the current iteration is 3, it would return 7$loop->count
Returns the total number of iterations or the total number of items in the array$loop->first
Returns true if it is the first iteration or item in the loop else returns false.$loop->last
Returns true if it is the last iteration or item in the loop else return false.$loop->depth
Returns the depth or nesting level of the current loop; returns 2 if it is a loop within a loop and 3 if it is nested one level more$loop->parent
If this loop is nested within another@foreach
loop,parent
returns the parent’s loop variable; If it is not tested, returns null
It is very easy to use and understand. So, you can do something like this in our view file:
@foreach($items as $item) @if($loop->first) <p>Our first element of the array</p> @endif <p>{{ $loop->iteration . '/' . $loop->count }}</p> @if($loop->last) <p>Our last element of the array</p> @endif @endforeach
You get a reference to the parent loop $loop
variable when you are in a nested loop. You can use depth
to determine the nesting level of the loop. Then, you can use parent
to get the $loop
variable of the parent. If you are nested further, you can chain the parent property again to get the $loop
variable of the parent of the parent. You can see in the code below, we have the depth of 3.
Let’s say we have the following array in the controller that we pass to our view.
$items = [ '1' => [ '1.1' => [ '1.1.1', '1.1.2' ], '1.2' => [ '1.2.1', '1.2.2' ] ], '2' => [ '2.1' => [ '2.1.1', '2.1.2' ], '2.2' => [ '2.2.1', '2.2.2' ] ] ];
Now, here is our code in the view using parent
to recursively go through the full array. This code is only for demonstration to show you that you can go up to any level of depth.
<ul> @foreach($items as $item => $value) <li>{{ $item }} == {{ $loop->iteration }} <ul> @foreach($value as $item_second => $value_second) <li>{{ $item_second }} == {{ $loop->parent->iteration }}.{{ $loop->iteration }} <ul> @foreach($value_second as $value_third) <li>{{ $value_third }} == {{ $loop->parent->parent->iteration }}. {{ $loop->parent->iteration }}.{{ $loop->iteration }} </li> @endforeach </ul> </li> @endforeach </ul> </li> @endforeach </ul>
The above code might look a little bit complex but we are just using the iteration
of the parent to create a pattern. Here is how the output looks:
Hopefully, you are now confident using $loop
variable in your projects.