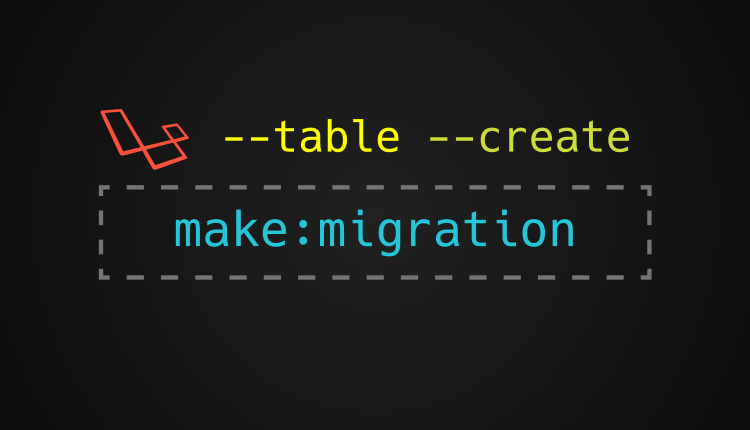
A quick little tip for guys who are new to Laravel. The artisan command make:migration
accepts optional parameters -create
and -table
. These parameters allows you to change generate stubs created with the migration file. Let’s check out the differences.
Table of Contents
Simple Migration Command
You can run the migration command without any parameters to create a simple migration file. Let’s create a posts table.
php artisan make:migration create_posts_table
This file outputs a simple migration file. The below output is just the class without any additional data.
class CreatePostsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->increments('id'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); } }
Parameter -create
Create parameter allows you to specify the name of the table. If you are using laravel convention, it uses the middle name from the name of the migration. Like from the create_posts_table
, it created migration for the posts table. So, if you want to create a new table but with the different name than used by laravel, you can specify a -create
parameter.
php artisan make:migration create_threads_table --create=replies
Above command creates a new migration file with the name create_threads_table
but with the table name of replies.
Here’s the contents of the above generated class.
class CreateThreadsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('replies', function (Blueprint $table) { $table->increments('id'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('replies'); } }
Parameter -table
Table parameter allows you to generate a migration file that will be used to alter the table. It is generally used to add a column to an already created table. We pass the name of the table in the -table
parameter which specifies the table to be altered. Let’s run this command to add an admin column to the users table. Below command is the convention mostly used by laravel community.
php artisan make:migration add_admin_field_to_users_table --table=users
The above command creates the class with the contents below.
class AddAdminFieldToUsersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('users', function (Blueprint $table) { // }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::table('users', function (Blueprint $table) { // }); } }
In this case, laravel does not provide a lot of help. We have to add the name of the column or whatever we want to change. In the down
method, you can also specify how to destroy that change.
That’s a simple tip that you can use in your projects.