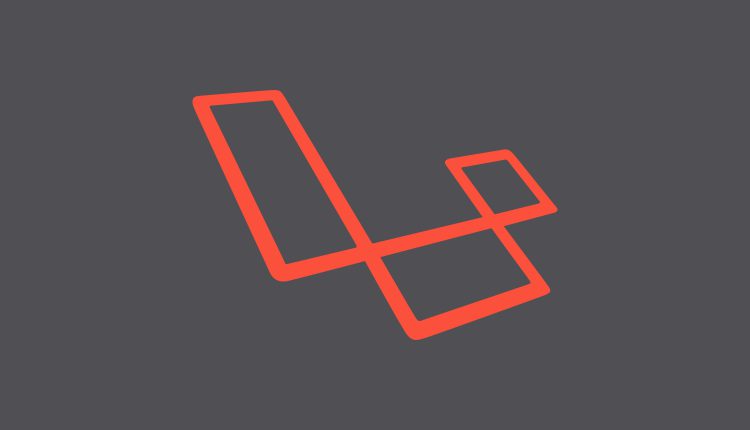
Creating your own PHP helper functions in Laravel
Laravel provides us with many built-in helper functions that you can call anywhere within your application. They make you workflow convenient for working with arrays & objects, paths, strings, URLs and other types.
Although there are many helper functions defined in the laravel core, you can define your own helper functions in laravel to avoid repeating the same code. It ensures better maintainability of your application.
Let’s walk through how you can create your own custom Laravel helper functions.
Table of Contents
Helpers in Laravel
There are many built-in helpers available in the laravel that you can use in your applications. They are grouped based on the type of functionality they provide. Here is a complete documentation of built-in laravel helpers.
Arrays & Objects
In this group, helpers provide the ability to work with arrays & objects. This group contains helper functions to add two arrays, collapse multidimensional array into a single array, return the first element of the array, check whether a given item or items exist in an array and perform many other types of manipulations.
Paths
This group of helpers returns absolute paths of different directories in your Laravel application like app, config, public, resource, storage and your application base path as well.
Strings
Helpers in this group work with string manipulation. You can convert strings to camel case, find the basename of the class, run htmlspecialchars, convert text to kebab case, convert text to snake case, and perform many other types of string manipulations.
URLs
URLs group of helpers works with generating URLs. You can generate URL for the controller action, named route, and fully qualified URL to the given path.
Miscellaneous
This category of helpers contains functions for working with page status, service container, authentication, cache etc.
Creating a Helper file in Laravel
In this section, we will go through creating a Laravel helper file that can be used globally in a Laravel application. You can organize the location of your helper file, however, I prefer to keep my Laravel project helper file in app/Helpers/Helper.php
. In this tutorial, we will create a helper file in my desired location.
Creating Helpers file
You could place your helper file anywhere in your Laravel application, it is standard to put it under your app
directory.
Let’s create a Helpers
directory under app
and create a Helper.php
file. These are the following contents of the file.
<?php if (!function_exists('human_file_size')) { /** * Returns a human readable file size * * @param integer $bytes * Bytes contains the size of the bytes to convert * * @param integer $decimals * Number of decimal places to be returned * * @return string a string in human readable format * * */ function human_file_size($bytes, $decimals = 2) { $sz = 'BKMGTPE'; $factor = (int)floor((strlen($bytes) - 1) / 3); return sprintf("%.{$decimals}f", $bytes / pow(1024, $factor)) . $sz[$factor]; } } if (!function_exists('in_arrayi')) { /** * Checks if a value exists in an array in a case-insensitive manner * * @param mixed $needle * The searched value * * @param $haystack * The array * * @param bool $strict [optional] * If set to true type of needle will also be matched * * @return bool true if needle is found in the array, * false otherwise */ function in_arrayi($needle, $haystack, $strict = false) { return in_array(strtolower($needle), array_map('strtolower', $haystack), $strict); } }
If you are using a class and its methods are your helpers you can start the file with namespace declaration.
namespace App\Helpers;
If you do not use the namespace declaration, these functions will become globally available and you can use them without even specifying the namespace. All the Laravel built-in helper functions are defined without a namespace. Also, helper class will also be available globally. Therefore, if you want to use helpers without specifying namespace simply remove this line.
There are a few caveats when defining these functions. All the Laravel helper files functions are rapped in a check to avoid function definition collisions.
if (!function_exists('human_file_size')) { function human_file_size($bytes, $decimals = 2) { // ... } }
If you skip this check, collisions will occur any time you redefine a function with the same definition. You could either use this check or you could also prefix your function names to avoid collisions.
Using the Helper file
Now, that’s it as far as our helper file is concerned. Let’s see how you can use helper file in Laravel application.
- You can either autoload helper file with composer. Then, you could use these functions anywhere in your application.
- You could also use the Laravel service provider to register this file. Laravel will load it along with other dependencies.
- You can also use a package that gives you all of this functionality.
Let’s see how we can use all of these methods.
Using Composer to Autoload Files
First one is pretty easy and straightforward. Simply go to composer.json file located in your Laravel project and you will see autoload key. Composer has a files key (an array of file paths that you want to autoload) that you can use in your autoload key. It will look like this:
"autoload": { "files": [ "app/Helpers/Helper.php" ], "classmap": [ "database/seeds", "database/factories" ], "psr-4": { "App\\": "app/" } },
After changing composer.json file and adding a new path to the files array, you need to dump the autoloader. Simply run this command from the terminal in your Laravel project directory.
composer dump-autoload
Now your helper file will be automatically loaded in your Laravel project.
Using service providers to load file
Let’s see how you can use service providers to autoload Helper file. Go to the command line in the root of your application and run the following command to create a new service provider.
php artisan make:provider HelperServiceProvider
You will see this message logged onto your console screen
Provider created successfully.
Once the service provider is created successfully, open that file. In the register method require your Helper files.
public function register() { $file = app_path('Helpers/Helper.php'); if (file_exists($file)) { require_once($file); } }
In the register method, we include our dependencies. In a large-scale project, it is possible that you have multiple helper files in a directory and you want to require all of them. You could change the register method like given below and your service provider will load all of the files in the Helpers directory.
public function register() { foreach (glob(app_path() . '/Helpers/*.php') as $file) { require_once($file); } }
It will require all of the files present in the app/Helpers
directory.
Now that our service provider is completed, we need to register our service provider, so, that Laravel will load it during bootstrapping. For this, go to config/app.php
and add the following line in the providers array at the end.
App\Providers\HelperServiceProvider::class,
If your helper file involves a class that has those helper methods and you have specified namespace, you could use them with little effort by defining an alias. You can do that easily by adding the following at the end of the aliases array in config/app.php
file.
'Helper' => App\Helpers\Helper::class,
By adding this in the alias array, you will be able to call your helpers by using the Helper
keyword. That’s all for creating your helpers with service providers.
Using a third party package
You could also use a third party package. Github page for the Laravel helpers package. You could install it via composer by running this command from the root of your application in console.
composer require browner12/helpers
Add the following line in the providers array in config/app.php
browner12\helpers\HelperServiceProvider::class,
If you are using Laravel’s automatic package discovery, you can skip this step.
After completing the necessary steps, you could use this command to create a Helper file.
php artisan make:helper Helper
It will create a Helper.php
file in App\Helpers
where you could easily add all of your helper functions.
Helper in Action
Now that we have our functions defined in our Helper file without a namespace defined, we could use them very easily. Simply go to your routes file at routes/web.php
and use this function for the home page route. For instance, this is the complete routes/web.php
file without comments:
<?php Route::get('/', function () { return human_file_size(1024*1024); });
It will simply return a human-readable size of the number of bytes passed as the parameter. You could call these functions from anywhere controllers or views.
Recommended Learning:
(2018) Career Hacking: Resume, LinkedIn, Interviewing +More
30,377 recommends, 4.4/5.0 stars and 2957 Ratings
Resources
Check out all of the built-in Laravel helpers available.
A simple package to create helper file browner12/helpers.
Learn more about using Composer Autoloader
Leave your comments, problems or queries in the feed below. I will respond to them as fast as I can.