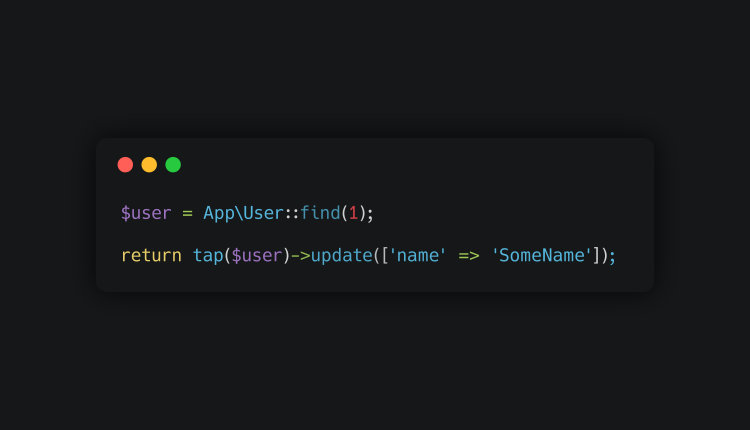
Laravel includes a variety of global helper functions. There are a ton of helper functions included with laravel that you can use to make your development workflow easier. Here, I will be writing 10 best laravel helpers that I use to make my development easier. You must consider using them where necessary as well.
You can also check out the official documentation for all laravel helper functions.
Table of Contents
array_dot()
The array_dot()
helper function allows you to convert a multidimensional array into a single dimensional that uses dot notation.
$array = [ 'user' => ['username' => 'something'], 'app' => ['creator' => ['name' => 'someone'], 'created' => 'today'] ]; $dot_array = array_dot($array); // [user.username] => something, [app.creator.name] => someone, [app.created] => today
array_get()
The array_get()
function retrieves the value from a multidimensional array using dot notation.
$array = [ 'user' => ['username' => 'something'], 'app' => ['creator' => ['name' => 'someone'], 'created' => 'today'] ]; $name = array_get($array, 'app.creator.name'); // someone
array_get()
function also accepts an optional third parameter to be used as the default value if the key is not present.
$name = array_get($array, 'app.created.name', 'anonymous'); // anonymous
public_path()
The public_path()
returns a fully qualified absolute path to the public
directory in your laravel application. You can also pass the path to a file or directory within the public directory to get the absolute path to that resource. It will simply append public_path()
to your argument.
$public_path = public_path(); $path = public_path('js/app.js');
Str::orderedUuid()
The Str::orderedUuid()
function generates a timestamp first uuid. This uuid can be stored in an indexed database column. These uuid’s created based on the timestamp, so they keep your content indexed. When using this in Laravel 5.6, it throws a Ramsey \ Uuid \ Exception \ UnsatisfiedDependencyException
. To fix this, simply require moontoast/math
package by running the following command:
composer require "moontoast/math"
use Illuminate\Support\Str; return (string) Str::orderByUuid() // A timestamp first uuid
str_plural()
The str_plural
function converts a string to its plural form. This function only supports the English language.
echo str_plural('bank'); // banks echo str_plural('developer'); // developers
route()
The route()
function generates a route URL for a named route.
$url = route('login');
If the route accepts parameters, you can simply pass them in an array as the second argument.
$url = route('products', ['id' => 1]);
If you want to generate a relative URL rather than an absolute URL, you can pass false
as the third argument.
$url = route('products', ['id' => 1], false);
tap()
The tap() function accepts two arguments: a value and a closure. The value will be passed to the closure and then the value will be returned. Closure return value does not matter.
$user = App\User::find(1); return tap($user, function($user) { $user->update([ 'name' => 'Random' ]); });
Instead of returning a boolean, it will return the user model.
If you do not pass closure, you can chain any methods on the value. The return will always be value
regardless what methods actually return. In the example below, it will return the user model instead of the boolean. Update method returns the boolean but because of the tap, it will return the user model.
$user = App\User::find(1); return tap($user)->update([ 'name' => 'SomeName' ]);
Learn more about Tap in laravel.
dump()
The dump() function dumps the given variables without stopping the execution. It is very useful for debugging.
dump($var1); dump($var1, $var2, $var3);
str_slug()
The str_slug()
function generates a URL friendly slug from the given string. You can use this function to create a slug for your post or product title.
$slug = str_slug('Helpers in Laravel', '-'); // helpers-in-laravel
optional()
The optional()
function accepts an argument and you can call its methods or access properties. If the passed object is null, methods and properties will return null instead of causing errors or throwing exceptions.
$user = User::find(1); return optional($user)->name;
Also, check out how to create your own helper functions in Laravel.
Find out top 10 best packages for extending Laravel.