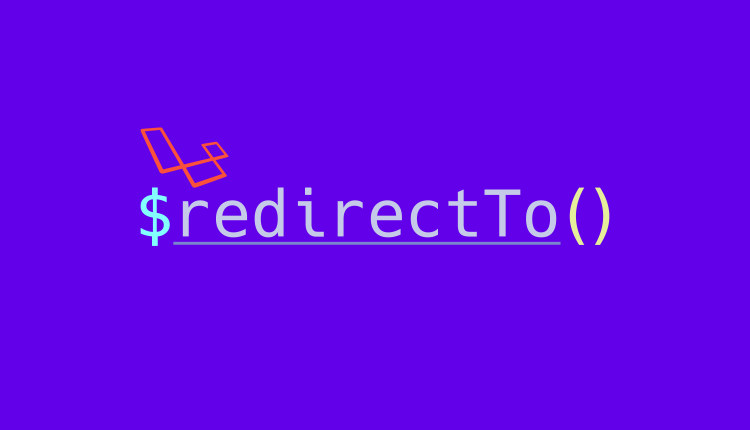
Laravel built-in authentication system has a $redirectTo
property that you can use to customize where the user will be redirected after successful login/registration. But we can customize even more than that.
By default, app/Http/Controllers/Auth/RegisterController.php
has the $redirectTo
property.
class RegisterController extends Controller { ... protected $redirectTo = '/home'; ... }
This means that the user will be redirected to /home
after successful registration.
Same property also exists in app/Http/Controllers/Auth/LoginController.php
which we can use to change where a user will be redirected after successful login.
What if we want to use different redirect path for different users based on certain conditions?
We can also use a method to add some logic to decide the redirect path for the user. We can define a method redirectTo
that will overwrite the $redirectTo
property.
class RegisterController extends Controller { public function redirectTo() { if (auth()->user()->is_admin) { return '/admin/dashboard'; } else if (auth()->user()->is_authenticated) { return '/app'; } else { return '/home'; } } }
We can do the same thing in LoginController.php
file as well to decide the return path after successful login.
Internal Implementation
Let’s see how it works internally. After every successful login/registration, laravel redirects to the path returned from redirectPath
method. This method is available in the trait RedirectsUsers
. This trait is implemented by both RegisterController.php
and LoginController.php
. This method works in this way. If the method redirectPath
is available, it calls the method and returns its return value. Otherwise, it simply returns the $redirectTo
property. Here is the actual trait file.
<?php namespace Illuminate\Foundation\Auth; trait RedirectsUsers { /** * Get the post register / login redirect path. * * @return string */ public function redirectPath() { if (method_exists($this, 'redirectTo')) { return $this->redirectTo(); } return property_exists($this, 'redirectTo') ? $this->redirectTo : '/home'; } }
Learn more about authentication in the laravel official documentation.